This project attempted to blend two "keyboards" in my house into one. The full Github project can be found here.
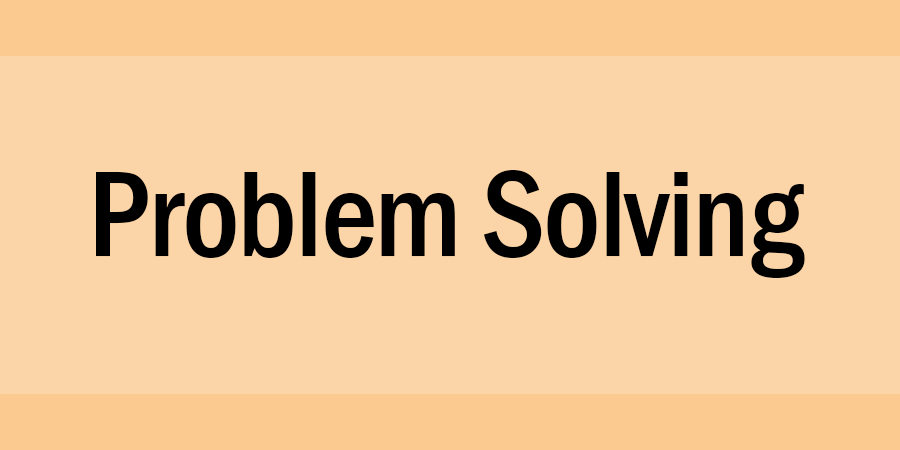
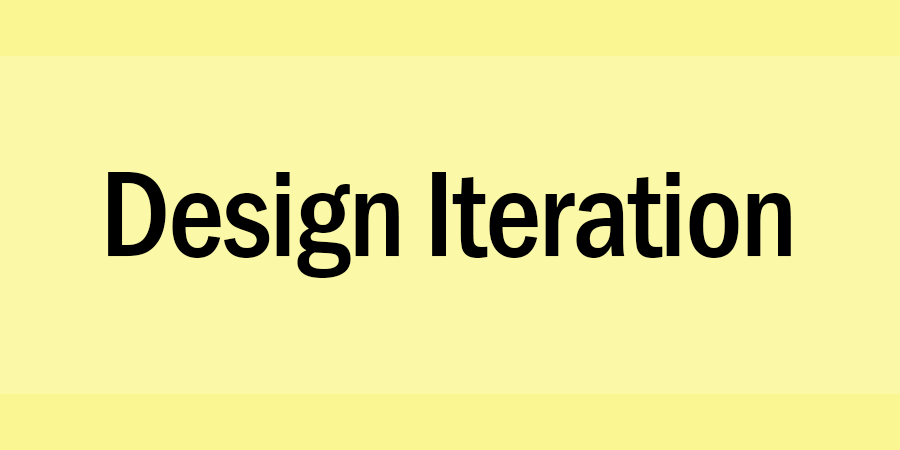
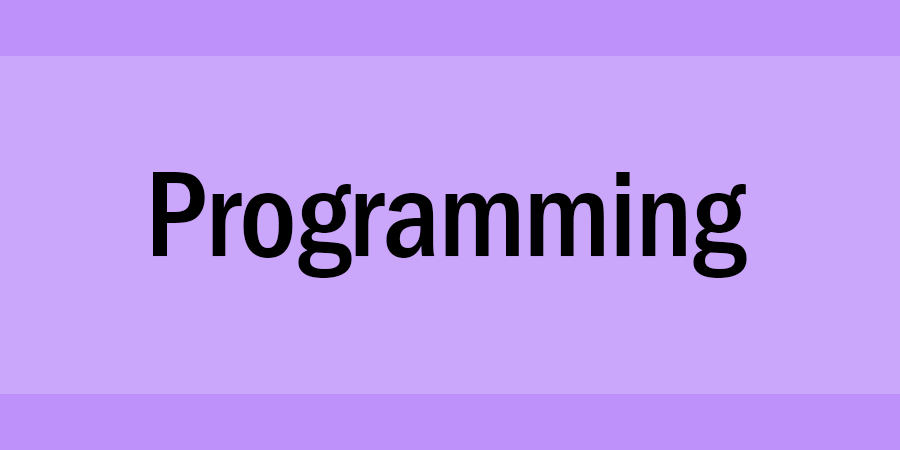
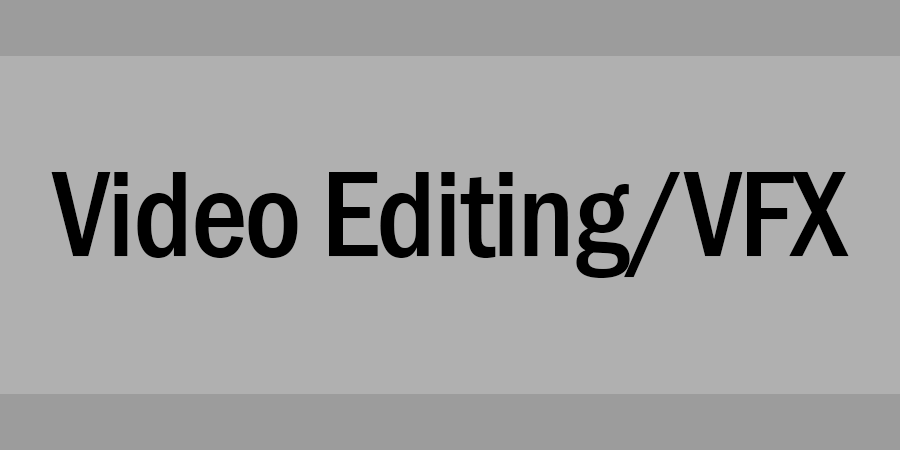
Goal
I wanted to create a program that would allow me to use my digital piano as my computer keyboard and make a visual digital representation of the keys I was pressing. I used Python to facilitate the interaction between the multiple systems I would be interfacing with.
Structure
Since I had an idea of what I wanted the interface to look like—and would allow me to have a frame of reference if the keystroke system was functional—I began by designing a simplistic piano representation that I could distribute programmatically to simulate octaves. I would then load and draw that image to a window, then overlay individually rendered keys on top of the base image to show them turning on or off. Through a USB connection, I could read the keyboard's MIDI events and detect keystrokes, which were then converted to piano events.
Obstacles
When I started the project, I theorized that I would need to do 4 main actions in the script in a loop:
1. Get all MIDI events from any connected keyboard.
2. Process these keystrokes into a more readable form.
3. Convert these piano keystrokes into computer keystrokes using a set of keybinds.
4. Update display based on new/held keystrokes.
Because of this, I believed that threading was the answer to this problem. I thought that the MIDI input would need to operate at a much faster rate than the display, which would not be able to keep up with a high clock speed. After setting up threading to isolate major components of the program, I realized I had created race conditions in my code that hampered the feasibility of keeping up-to-date lists of held keys, so I decided to restructure to a more linear approach.
The new linear approach involved reading and writing midi data, toggling devices, and updating the display all within the same logic loop. This meant there was little to no information passing between threads since now, the display object also contained the held key information.
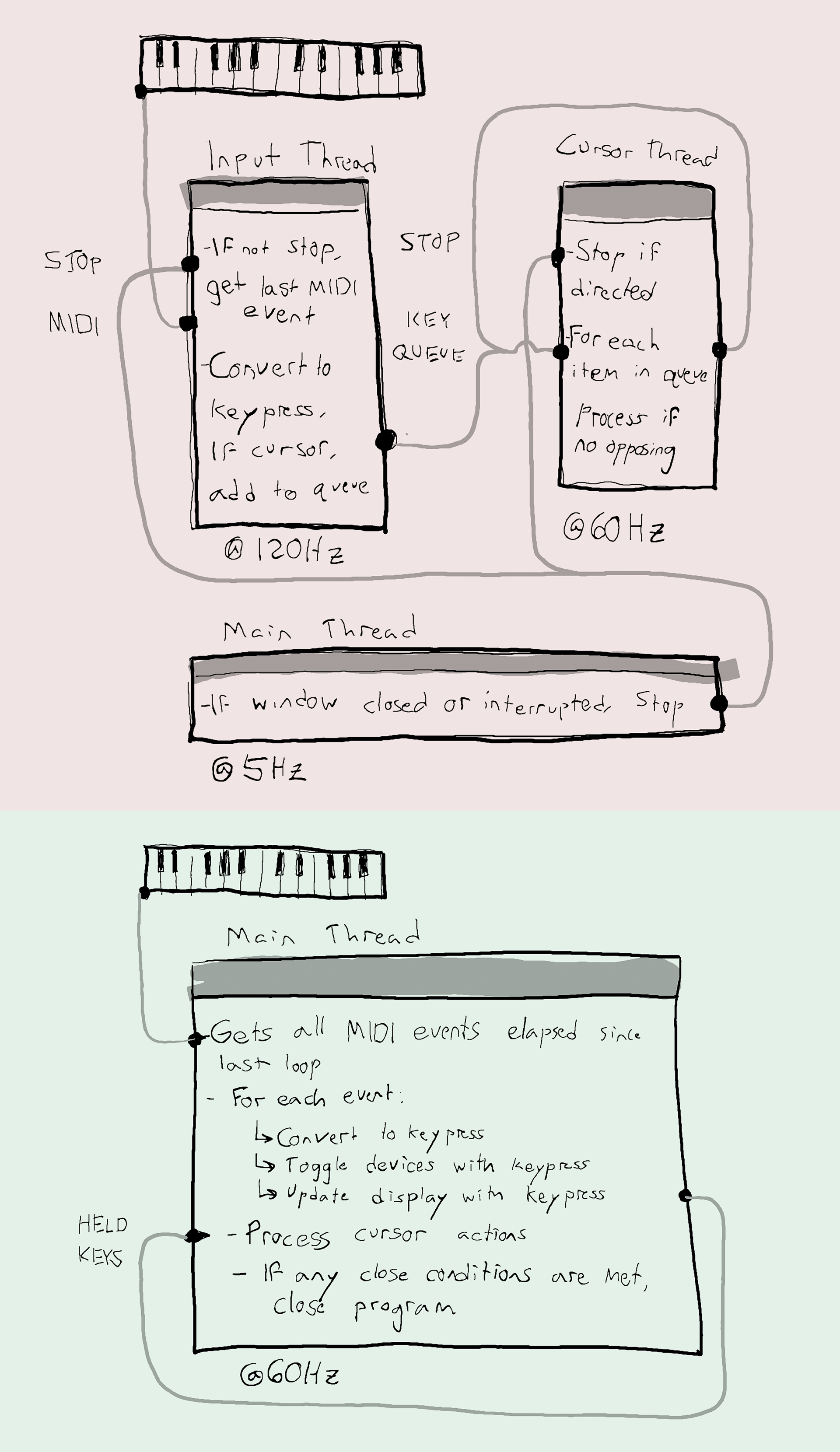
Animation
Lastly, I created a script that would allow me to create custom animations on the display, to be driven by keyframing or mathematical sequencing.
